Mysql_fetch_array() returns row as an associative, numeric array or both based on parameters passed in it. Three types of parameters can be passed in mysql_fetch_array function.
1. MYSQL_BOTH - return both associative and numeric array
2. MYSQL_ASSOC - return associative array
3. MYSQL_NUM - return numeric array
Consider following example:
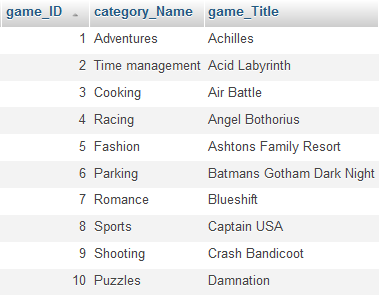
Now you'll get output like this:
Where,
the array should be like this:
Array(
[game_ID] => 1
[category_Name] => Adventures
[game_Title] => Achilles
)
Now you'll get output like this:
In this type, the array of result should be like below:
Array(
[0] => 1
[1] => Adventures
[2] => Achilles
)
Suppose you'll use
echo'<tr><td>'.$res[0].'</td><td>'.$res[1].'</td><td>'.$res['game_Title'].'</td></tr>';
That means you use associative index instead numerical, then you will get undefined index error like as follows as:
Where,
the array should be like this:
Array(
[game_ID] => 1
[category_Name] => Adventures
[game_Title] => Achilles
)
That means you use numeric index instead associative, then you will get undefined offset error like as follows as:
Note:
1. MYSQL_BOTH - return both associative and numeric array
2. MYSQL_ASSOC - return associative array
3. MYSQL_NUM - return numeric array
Retrieve data from table using mysql_fetch_array() in php:
Consider following example:
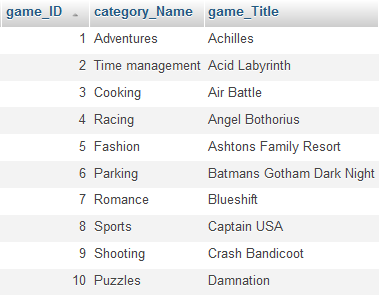
Mysql_fetch_array() with MYSQL_BOTH in php:
It returns result as both associative and numeric array. You can retrieve data using both associative and numeric indices in php.
<html>
<body>
<table>
<th>Game_ID</th><th>Category</th><th>Title</th>
<?php
mysql_connect('localhost','root','') or die(mysql_error());
mysql_select_db('new') or die(mysql_error());
$query=mysql_query('select * from table1') or die(mysql_error());
while($res=mysql_fetch_array($query, MYSQL_BOTH))
{
echo'<tr><td>'.$res['game_ID'].'</td><td>'.$res[1].'</td><td>'.$res['game_Title'].'</td></tr>';
}
echo'<table>';
?>
</body>
</html>
<body>
<table>
<th>Game_ID</th><th>Category</th><th>Title</th>
<?php
mysql_connect('localhost','root','') or die(mysql_error());
mysql_select_db('new') or die(mysql_error());
$query=mysql_query('select * from table1') or die(mysql_error());
while($res=mysql_fetch_array($query, MYSQL_BOTH))
{
echo'<tr><td>'.$res['game_ID'].'</td><td>'.$res[1].'</td><td>'.$res['game_Title'].'</td></tr>';
}
echo'<table>';
?>
</body>
</html>
Now you'll get output like this:
Where,
the array should be like this:
Array(
[game_ID] => 1
[category_Name] => Adventures
[game_Title] => Achilles
)
OR
Array(
[0] => 1
[1] => Adventures
[2] => Achilles
)
[0] => 1
[1] => Adventures
[2] => Achilles
)
mysql_fetch_array() with MYSQL_NUM in php:
It returns result as numeric array. ie, indices are numeric like 0,1,2...
<html>
<body>
<table>
<th>Game_ID</th><th>Category</th><th>Title</th>
<?php
mysql_connect('localhost','root','') or die(mysql_error());
mysql_select_db('new') or die(mysql_error());
$query=mysql_query('select * from table1') or die(mysql_error());
while($res=mysql_fetch_array($query, MYSQL_NUM))
{
echo'<tr><td>'.$res[0].'</td><td>'.$res[1].'</td><td>'.$res[2].'</td></tr>';
}
echo'<table>';
?>
</body>
</html>
<body>
<table>
<th>Game_ID</th><th>Category</th><th>Title</th>
<?php
mysql_connect('localhost','root','') or die(mysql_error());
mysql_select_db('new') or die(mysql_error());
$query=mysql_query('select * from table1') or die(mysql_error());
while($res=mysql_fetch_array($query, MYSQL_NUM))
{
echo'<tr><td>'.$res[0].'</td><td>'.$res[1].'</td><td>'.$res[2].'</td></tr>';
}
echo'<table>';
?>
</body>
</html>
Now you'll get output like this:
In this type, the array of result should be like below:
Array(
[0] => 1
[1] => Adventures
[2] => Achilles
)
Suppose you'll use
echo'<tr><td>'.$res[0].'</td><td>'.$res[1].'</td><td>'.$res['game_Title'].'</td></tr>';
That means you use associative index instead numerical, then you will get undefined index error like as follows as:
mysql_fetch_array() with MYSQL_ASSOC in php:
It returns result as an associative array.
<html>
<body>
<table>
<th>Game_ID</th><th>Category</th><th>Title</th>
<?php
mysql_connect('localhost','root','') or die(mysql_error());
mysql_select_db('new') or die(mysql_error());
$query=mysql_query('select * from table1') or die(mysql_error());
while($res=mysql_fetch_array($query,MYSQL_ASSOC))
{
echo'<tr><td>'.$res['game_ID'].'</td><td>'.$res['category_Name'].'</td><td>'.$res['game_Title'].'</td></tr>';
}
echo'<table>';
?>
</body>
</html>
<body>
<table>
<th>Game_ID</th><th>Category</th><th>Title</th>
<?php
mysql_connect('localhost','root','') or die(mysql_error());
mysql_select_db('new') or die(mysql_error());
$query=mysql_query('select * from table1') or die(mysql_error());
while($res=mysql_fetch_array($query,MYSQL_ASSOC))
{
echo'<tr><td>'.$res['game_ID'].'</td><td>'.$res['category_Name'].'</td><td>'.$res['game_Title'].'</td></tr>';
}
echo'<table>';
?>
</body>
</html>
Now you'll get output like this:
Where,
the array should be like this:
Array(
[game_ID] => 1
[category_Name] => Adventures
[game_Title] => Achilles
)
Suppose you'll use
echo'<tr><td>'.$res[0].'</td><td>'.$res['category_Name'].'</td><td>'.$res['game_Title'].'</td></tr>';
That means you use numeric index instead associative, then you will get undefined offset error like as follows as:
Note:
mysql_fetch_array() without array_type means return row as an associative, numeric array or both
Related Post:
Related Post:
No comments:
Post a Comment